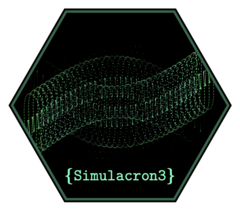
Simulation R6Class
Simulation.Rd
Takes a specification for a data generating process (dgp
),
list of estimators
, config
, list of summary_statistics
to compute, and
provides a get_results
method.
Details
The core idea is that a statistical simulation study consists of specifying a repeatable data generating process, some functions (estimators) to run on each generated data sample, and some summary statistics to compute from the simulation results (typically that indicate aspects of the performance of the estimators considered). This is represented by the following pipeline:
Public fields
dgp
A function that takes a single argument
n
for sample size and generates synthetic data.estimators
A list of estimators that each can be called on the data
config
A list containing at least the number of
replications
to perform, thesample_size
to use, and whether or not to run inparallel
.summary_stats
A list of summary statistic functions that can be called on the estimates produced
results
A data.frame of results from running the simulation
initialize
Method to initialize the simulation object (does nothing)
set_dgp
Method to set the data generating process
set_estimators
Method to set the estimators
set_config
Method to set the configuration
get_results
Method to retrieve results
set_summary_stats
Method to set summary statistics
run
Method to run the simulation
Active bindings
initialize
Method to initialize the simulation object (does nothing)
set_dgp
Method to set the data generating process
set_estimators
Method to set the estimators
set_config
Method to set the configuration
get_results
Method to retrieve results
set_summary_stats
Method to set summary statistics
run
Method to run the simulation
Methods
Method new()
Usage
Simulation$new()
Examples
if (FALSE) { # \dontrun{
# Example Usage
# Define a data generating process
dgp <- function(n) data.frame(x = rnorm(n), y = rnorm(n))
# Define some estimators
estimators <- list(
mean_estimator = function(data) mean(data$x),
var_estimator = function(data) var(data$x)
)
# Define a summary statistics function
summary_func <- function(iter = NULL, est_results, data = NULL) {
data.frame(
mean_est = est_results$mean_estimator,
var_est = est_results$var_estimator
)
}
# Create a simulation object
sim <- Simulation$new()
# Set up the simulation
sim$set_dgp(dgp)
sim$set_estimators(estimators)
sim$set_config(list(replications = 500, sample_size = 50))
sim$set_summary_stats(summary_func)
# Run the simulation
sim$run()
# Retrieve results
results <- sim$get_results()
head(results)
} # }